Cron is a time-based job scheduler in Unix-like computer operating systems. The name cron comes from the word "chronos", Greek for "time". Cron enables users to schedule jobs (commands or shell scripts) to run periodically at certain times or dates. It is commonly used to automate system maintenance or administration, though its general-purpose nature means that it can be used for other purposes, such as connecting to the Internet and downloading email.[1]
Overview
Cron is driven by a crontab, a configuration file that specifies shell commands to run periodically on a given schedule. The crontab files are stored where the lists of jobs and other instructions to the cron daemon are kept. Users can have their own individual crontab files and often there is a systemwide crontab file (usually in /etc or a subdirectory of /etc) which only system administrators can edit.
Each line of a crontab file represents a job and is composed of a CRON expression, followed by a shell command to execute. Some implementations of cron, such as that in the popular 4th BSD edition written by Paul Vixie and included in many Linux distributions, add a username specification into the format as the sixth field, as whom the specified job will be run (subject to user existence in /etc/passwd and allowed permissions). This is only allowed in the system crontabs (/etc/crontab and /etc/cron.d/*), not in others which are each assigned to a single user to configure.
For "day of the week" (field 5), both 0 and 7 are considered Sunday, though some versions of Unix such as AIX do not list "7" as acceptable in the man page. While normally the job is executed when the time/date specification fields all match the current time and date, there is one exception: if both "day of month" and "day of week" are restricted (not "*"), then either the "day of month" field (3) or the "day of week" field (5) must match the current day.
[edit] Examples
The following will clear the Apache error log at one minute past midnight (00:01 of every day of the month, of every day of the week).
1 0 * * * echo -n "" > /www/apache/logs/error_log
The following will run the script /home/user/test.pl every 5 minutes.
*/5 * * * * /home/user/test.pl
.---------------- minute (0 - 59)
| .------------- hour (0 - 23)
| | .---------- day of month (1 - 31)
| | | .------- month (1 - 12) OR jan,feb,mar,apr ...
| | | | .---- day of week (0 - 7) (Sunday=0 or 7) OR sun,mon,tue,wed,thu,fri,sat
| | | | |
* * * * * command to be executed
Predefined scheduling definitions
There are several special predefined values which can be used to substitute the CRON expression.
Entry Description Equivalent To
@yearly Run once a year 0 0 1 1 *
@annually (same as @yearly) 0 0 1 1 *
@monthly Run once a month 0 0 1 * *
@weekly Run once a week 0 0 * * 0
@daily Run once a day 0 0 * * *
@midnight (same as @daily) 0 0 * * *
@hourly Run once an hour 0 * * * *
This blog is made for Expressing My Ideas, Views, and to put some technical datas.
Monday, May 17, 2010
Saturday, May 15, 2010
Data structure alignment
Data structure alignment is the way data is arranged and accessed in computer memory. It consists of two separate but related issues: data alignment and data structure padding. When a modern computer reads from or writes to a memory address, it will do this in word sized chunks (e.g. 4 byte chunks on a 32-bit system). Data alignment means putting the data at a memory offset equal to some multiple of the word size, which increases the system's performance due to the way the CPU handles memory. To align the data, it may be necessary to insert some meaningless bytes between the end of the last data structure and the start of the next, which is data structure padding.
For example, when the computer's word size is 4 bytes, the data to be read should be at a memory offset which is some multiple of 4. When this is not the case, e.g. the data starts at the 14th byte instead of the 16th byte, then the computer has to read two 4-byte chunks and do some calculation before the requested data has been read, or it may generate an alignment fault. Even though the previous data structure ends at the 14th byte, the next data structure should start at the 16th byte. Two padding bytes are inserted between the two data structures to align the next data structure to the 16th byte
Typical alignment of C structs on x86
Data structure members are stored sequentially in a memory so that in the structure below the member Data1 will always precede Data2 and Data2 will always precede Data3:
struct MyData
{
short Data1;
short Data2;
short Data3;
};
If the type "short" is stored in two bytes of memory then each member of the data structure depicted above would be 2-byte aligned. Data1 would be at offset 0, Data2 at offset 2 and Data3 at offset 4. The size of this structure would be 6 bytes.
The type of each member of the structure usually has a default alignment, meaning that it will, unless otherwise requested by the programmer, be aligned on a pre-determined boundary. The following typical alignments are valid for compilers from Microsoft, Borland, and GNU when compiling for 32-bit x86:
* A char (one byte) will be 1-byte aligned.
* A short (two bytes) will be 2-byte aligned.
* An int (four bytes) will be 4-byte aligned.
* A float (four bytes) will be 4-byte aligned.
* A double (eight bytes) will be 8-byte aligned on Windows and 4-byte aligned on Linux.
* A long double (twelve bytes) will be 4-byte aligned on Linux.
* Any pointer (four bytes) will be 4-byte aligned on Linux. (e.g.: char*, int*)
The only notable difference in alignment for a 64-bit Linux system when compared to a 32 bit is:
* A double (eight bytes) will be 8-byte aligned.
* A long double (Sixteen bytes) will be 16-byte aligned.
* Any pointer (eight bytes) will be 8-byte aligned.
Here is a structure with members of various types, totaling 8 bytes before compilation:
struct MixedData
{
char Data1;
short Data2;
int Data3;
char Data4;
};
After compilation the data structure will be supplemented with padding bytes to ensure a proper alignment for each of its members:
struct MixedData /* after compilation */
{
char Data1;
char Padding1[1]; /* For the following 'short' to be aligned on a 2 byte boundary */
short Data2;
int Data3;
char Data4;
char Padding2[3];
};
The compiled size of the structure is now 12 bytes. It is important to note that the last member is padded with the number of bytes required to conform to the largest type of the structure. In this case 3 bytes are added to the last member to pad the structure to the size of a long word.
It is possible to change the alignment of structures to reduce the memory they require (or to conform to an existing format) by reordering structure members or changing the compiler’s alignment (or “packing”) of structure members.
struct MixedData /* after reordering */
{
char Data1;
char Data4; /* reordered */
short Data2;
int Data3;
};
The compiled size of the structure now matches the pre-compiled size of 8 bytes. Note that Padding1[1] has been replaced (and thus eliminated) by Data4 and Padding2[3] is no longer necessary as the structure is already aligned to the size of a long word.
The alternative method of enforcing the MixedData structure to be aligned to a one byte boundary will cause the pre-processor to discard the pre-determined alignment of the structure members and thus no padding bytes would be inserted.
Thanks to wikipedia:
http://en.wikipedia.org/wiki/Data_structure_alignment
For example, when the computer's word size is 4 bytes, the data to be read should be at a memory offset which is some multiple of 4. When this is not the case, e.g. the data starts at the 14th byte instead of the 16th byte, then the computer has to read two 4-byte chunks and do some calculation before the requested data has been read, or it may generate an alignment fault. Even though the previous data structure ends at the 14th byte, the next data structure should start at the 16th byte. Two padding bytes are inserted between the two data structures to align the next data structure to the 16th byte
Typical alignment of C structs on x86
Data structure members are stored sequentially in a memory so that in the structure below the member Data1 will always precede Data2 and Data2 will always precede Data3:
struct MyData
{
short Data1;
short Data2;
short Data3;
};
If the type "short" is stored in two bytes of memory then each member of the data structure depicted above would be 2-byte aligned. Data1 would be at offset 0, Data2 at offset 2 and Data3 at offset 4. The size of this structure would be 6 bytes.
The type of each member of the structure usually has a default alignment, meaning that it will, unless otherwise requested by the programmer, be aligned on a pre-determined boundary. The following typical alignments are valid for compilers from Microsoft, Borland, and GNU when compiling for 32-bit x86:
* A char (one byte) will be 1-byte aligned.
* A short (two bytes) will be 2-byte aligned.
* An int (four bytes) will be 4-byte aligned.
* A float (four bytes) will be 4-byte aligned.
* A double (eight bytes) will be 8-byte aligned on Windows and 4-byte aligned on Linux.
* A long double (twelve bytes) will be 4-byte aligned on Linux.
* Any pointer (four bytes) will be 4-byte aligned on Linux. (e.g.: char*, int*)
The only notable difference in alignment for a 64-bit Linux system when compared to a 32 bit is:
* A double (eight bytes) will be 8-byte aligned.
* A long double (Sixteen bytes) will be 16-byte aligned.
* Any pointer (eight bytes) will be 8-byte aligned.
Here is a structure with members of various types, totaling 8 bytes before compilation:
struct MixedData
{
char Data1;
short Data2;
int Data3;
char Data4;
};
After compilation the data structure will be supplemented with padding bytes to ensure a proper alignment for each of its members:
struct MixedData /* after compilation */
{
char Data1;
char Padding1[1]; /* For the following 'short' to be aligned on a 2 byte boundary */
short Data2;
int Data3;
char Data4;
char Padding2[3];
};
The compiled size of the structure is now 12 bytes. It is important to note that the last member is padded with the number of bytes required to conform to the largest type of the structure. In this case 3 bytes are added to the last member to pad the structure to the size of a long word.
It is possible to change the alignment of structures to reduce the memory they require (or to conform to an existing format) by reordering structure members or changing the compiler’s alignment (or “packing”) of structure members.
struct MixedData /* after reordering */
{
char Data1;
char Data4; /* reordered */
short Data2;
int Data3;
};
The compiled size of the structure now matches the pre-compiled size of 8 bytes. Note that Padding1[1] has been replaced (and thus eliminated) by Data4 and Padding2[3] is no longer necessary as the structure is already aligned to the size of a long word.
The alternative method of enforcing the MixedData structure to be aligned to a one byte boundary will cause the pre-processor to discard the pre-determined alignment of the structure members and thus no padding bytes would be inserted.
Thanks to wikipedia:
http://en.wikipedia.org/wiki/Data_structure_alignment
Thursday, May 13, 2010
Missing .emacs file
Missing emacs init file
you can't find your .emacs file anywhere in your system. You've already looked in you home directory and it's nowhere to be found. Could somebody help me out?
So the solution is here.
There are two main versions of emacs - emacs and Xemacs and I don't think that either of them creates a .emacs file automatically - you have to create your own.
So what do you put in your .emacs? There are lots of examples of .emacs's that you can google for on the net, but here's one to start you off...
http://www.dotemacs.de/dotfiles/sample.emacs.html
...just save it to .emacs in your home directory.
If you have Xemacs installed, they don't use a file called .emacs but create a directory called .xemacs. Inside that directory you create a file called custom.el and that functions as your .emacs.
location of .emacs file :
/home/yourname/.emacs
eg: /home/siva/.emacs
Then add according to your need of your choice in emacs and save the file
sample .emacs file
;; -*- Mode: Emacs-Lisp -*-
;;; This is a sample .emacs file.
;;;
;;; The .emacs file, which should reside in your home directory, allows you to
;;; customize the behavior of Emacs. In general, changes to your .emacs file
;;; will not take effect until the next time you start up Emacs. You can load
;;; it explicitly with `M-x load-file RET ~/.emacs RET'.
;;;
;;; There is a great deal of documentation on customization in the Emacs
;;; manual. You can read this manual with the online Info browser: type
;;; `C-h i' or select "Emacs Info" from the "Help" menu.
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Basic Customization ;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Enable the command `narrow-to-region' ("C-x n n"), a useful
;; command, but possibly confusing to a new user, so it's disabled by
;; default.
(put 'narrow-to-region 'disabled nil)
;;; Define a variable to indicate whether we're running XEmacs/Lucid Emacs.
;;; (You do not have to defvar a global variable before using it --
;;; you can just call `setq' directly like we do for `emacs-major-version'
;;; below. It's clearer this way, though.)
(defvar running-xemacs (string-match "XEmacs\\|Lucid" emacs-version))
;; Make the sequence "C-x w" execute the `what-line' command,
;; which prints the current line number in the echo area.
(global-set-key "\C-xw" 'what-line)
;; set up the function keys to do common tasks to reduce Emacs pinky
;; and such.
;; Make F1 invoke help
(global-set-key [f1] 'help-command)
;; Make F2 be `undo'
(global-set-key [f2] 'undo)
;; Make F3 be `find-file'
;; Note: it does not currently work to say
;; (global-set-key 'f3 "\C-x\C-f")
;; The reason is that macros can't do interactive things properly.
;; This is an extremely longstanding bug in Emacs. Eventually,
;; it will be fixed. (Hopefully ..)
(global-set-key [f3] 'find-file)
;; Make F4 be "mark", F5 be "copy", F6 be "paste"
;; Note that you can set a key sequence either to a command or to another
;; key sequence.
(global-set-key [f4] 'set-mark-command)
(global-set-key [f5] "\M-w")
(global-set-key [f6] "\C-y")
;; Shift-F4 is "pop mark off of stack"
(global-set-key [(shift f4)] (lambda () (interactive) (set-mark-command t)))
;; Make F7 be `save-buffer'
(global-set-key [f7] 'save-buffer)
;; Make F8 be "start macro", F9 be "end macro", F10 be "execute macro"
(global-set-key [f8] 'start-kbd-macro)
(global-set-key [f9] 'end-kbd-macro)
(global-set-key [f10] 'call-last-kbd-macro)
;; Here's an alternative binding if you don't use keyboard macros:
;; Make F8 be `save-buffer' followed by `delete-window'.
;;(global-set-key 'f8 "\C-x\C-s\C-x0")
;; If you prefer delete to actually delete forward then you want to
;; uncomment the next line (or use `Customize' to customize this).
;; (setq delete-key-deletes-forward t)
(cond (running-xemacs
;;
;; Code for any version of XEmacs/Lucid Emacs goes here
;;
;; Change the values of some variables.
;; (t means true; nil means false.)
;;
;; Use the "Describe Variable..." option on the "Help" menu
;; to find out what these variables mean.
(setq find-file-use-truenames nil
find-file-compare-truenames t
minibuffer-confirm-incomplete t
complex-buffers-menu-p t
next-line-add-newlines nil
mail-yank-prefix "> "
kill-whole-line t
)
;; When running ispell, consider all 1-3 character words as correct.
(setq ispell-extra-args '("-W" "3"))
(cond ((or (not (fboundp 'device-type))
(equal (device-type) 'x))
;; Code which applies only when running emacs under X goes here.
;; (We check whether the function `device-type' exists
;; before using it. In versions before 19.12, there
;; was no such function. If it doesn't exist, we
;; simply assume we're running under X -- versions before
;; 19.12 only supported X.)
;; Remove the binding of C-x C-c, which normally exits emacs.
;; It's easy to hit this by mistake, and that can be annoying.
;; Under X, you can always quit with the "Exit Emacs" option on
;; the File menu.
(global-set-key "\C-x\C-c" nil)
;; Uncomment this to enable "sticky modifier keys" in 19.13
;; and up. With sticky modifier keys enabled, you can
;; press and release a modifier key before pressing the
;; key to be modified, like how the ESC key works always.
;; If you hold the modifier key down, however, you still
;; get the standard behavior. I personally think this
;; is the best thing since sliced bread (and a *major*
;; win when it comes to reducing Emacs pinky), but it's
;; disorienting at first so I'm not enabling it here by
;; default.
;;(setq modifier-keys-are-sticky t)
;; This changes the variable which controls the text that goes
;; in the top window title bar. (However, it is not changed
;; unless it currently has the default value, to avoid
;; interfering with a -wn command line argument I may have
;; started emacs with.)
(if (equal frame-title-format "%S: %b")
(setq frame-title-format
(concat "%S: " invocation-directory invocation-name
" [" emacs-version "]"
(if nil ; (getenv "NCD")
""
" %b"))))
;; If we're running on display 0, load some nifty sounds that
;; will replace the default beep. But if we're running on a
;; display other than 0, which probably means my NCD X terminal,
;; which can't play digitized sounds, do two things: reduce the
;; beep volume a bit, and change the pitch of the sound that is
;; made for "no completions."
;;
;; (Note that sampled sounds only work if XEmacs was compiled
;; with sound support, and we're running on the console of a
;; Sparc, HP, or SGI machine, or on a machine which has a
;; NetAudio server; otherwise, you just get the standard beep.)
;;
;; (Note further that changing the pitch and duration of the
;; standard beep only works with some X servers; many servers
;; completely ignore those parameters.)
;;
(cond ((string-match ":0" (getenv "DISPLAY"))
(load-default-sounds))
(t
(setq bell-volume 40)
(setq sound-alist
(append sound-alist '((no-completion :pitch 500))))
))
;; Make `C-x C-m' and `C-x RET' be different (since I tend
;; to type the latter by accident sometimes.)
(define-key global-map [(control x) return] nil)
;; Change the pointer used when the mouse is over a modeline
(set-glyph-image modeline-pointer-glyph "leftbutton")
;; Change the continuation glyph face so it stands out more
(and (fboundp 'set-glyph-property)
(boundp 'continuation-glyph)
(set-glyph-property continuation-glyph 'face 'bold))
;; Change the pointer used during garbage collection.
;;
;; Note that this pointer image is rather large as pointers go,
;; and so it won't work on some X servers (such as the MIT
;; R5 Sun server) because servers may have lamentably small
;; upper limits on pointer size.
;;(if (featurep 'xpm)
;; (set-glyph-image gc-pointer-glyph
;; (expand-file-name "trash.xpm" data-directory)))
;; Here's another way to do that: it first tries to load the
;; pointer once and traps the error, just to see if it's
;; possible to load that pointer on this system; if it is,
;; then it sets gc-pointer-glyph, because we know that
;; will work. Otherwise, it doesn't change that variable
;; because we know it will just cause some error messages.
(if (featurep 'xpm)
(let ((file (expand-file-name "recycle.xpm" data-directory)))
(if (condition-case error
;; check to make sure we can use the pointer.
(make-image-instance file nil
'(pointer))
(error nil)) ; returns nil if an error occurred.
(set-glyph-image gc-pointer-glyph file))))
(when (featurep 'menubar)
;; Add `dired' to the File menu
(add-menu-button '("File") ["Edit Directory" dired t])
;; Here's a way to add scrollbar-like buttons to the menubar
(add-menu-button nil ["Top" beginning-of-buffer t])
(add-menu-button nil ["<<<" scroll-down t])
(add-menu-button nil [" . " recenter t])
(add-menu-button nil [">>>" scroll-up t])
(add-menu-button nil ["Bot" end-of-buffer t]))
;; Change the behavior of mouse button 2 (which is normally
;; bound to `mouse-yank'), so that it inserts the selected text
;; at point (where the text cursor is), instead of at the
;; position clicked.
;;
;; Note that you can find out what a particular key sequence or
;; mouse button does by using the "Describe Key..." option on
;; the Help menu.
(setq mouse-yank-at-point t)
;; When editing C code (and Lisp code and the like), I often
;; like to insert tabs into comments and such. It gets to be
;; a pain to always have to use `C-q TAB', so I set up a more
;; convenient binding. Note that this does not work in
;; TTY frames, where tab and shift-tab are indistinguishable.
(define-key global-map '(shift tab) 'self-insert-command)
;; LISPM bindings of Control-Shift-C and Control-Shift-E.
;; Note that "\C-C" means Control-C, not Control-Shift-C.
;; To specify shifted control characters, you must use the
;; more verbose syntax used here.
(define-key emacs-lisp-mode-map '(control C) 'compile-defun)
(define-key emacs-lisp-mode-map '(control E) 'eval-defun)
;; If you like the FSF Emacs binding of button3 (single-click
;; extends the selection, double-click kills the selection),
;; uncomment the following:
;; Under 19.13, the following is enough:
;(define-key global-map 'button3 'mouse-track-adjust)
;; But under 19.12, you need this:
;(define-key global-map 'button3
; (lambda (event)
; (interactive "e")
; (let ((default-mouse-track-adjust t))
; (mouse-track event))))
;; Under both 19.12 and 19.13, you also need this:
;(add-hook 'mouse-track-click-hook
; (lambda (event count)
; (if (or (/= (event-button event) 3)
; (/= count 2))
; nil ;; do the normal operation
; (kill-region (point) (mark))
; t ;; don't do the normal operations.
; )))
))
))
;; Oh, and here's a cute hack you might want to put in the sample .emacs
;; file: it changes the color of the window if it's not on the local
;; machine, or if it's running as root:
;; local emacs background: whitesmoke
;; remote emacs background: palegreen1
;; root emacs background: coral2
(cond
((and (string-match "XEmacs" emacs-version)
(eq window-system 'x)
(boundp 'emacs-major-version)
(= emacs-major-version 19)
(>= emacs-minor-version 12))
(let* ((root-p (eq 0 (user-uid)))
(dpy (or (getenv "DISPLAY") ""))
(remote-p (not
(or (string-match "^\\(\\|unix\\|localhost\\):" dpy)
(let ((s (system-name)))
(if (string-match "\\.\\(netscape\\|mcom\\)\\.com" s)
(setq s (substring s 0 (match-beginning 0))))
(string-match (concat "^" (regexp-quote s)) dpy)))))
(bg (cond (root-p "coral2")
(remote-p "palegreen1")
(t nil))))
(cond (bg
(let ((def (color-name (face-background 'default)))
(faces (face-list)))
(while faces
(let ((obg (face-background (car faces))))
(if (and obg (equal def (color-name obg)))
(set-face-background (car faces) bg)))
(setq faces (cdr faces)))))))))
;;; Older versions of emacs did not have these variables
;;; (emacs-major-version and emacs-minor-version.)
;;; Let's define them if they're not around, since they make
;;; it much easier to conditionalize on the emacs version.
(if (and (not (boundp 'emacs-major-version))
(string-match "^[0-9]+" emacs-version))
(setq emacs-major-version
(string-to-int (substring emacs-version
(match-beginning 0) (match-end 0)))))
(if (and (not (boundp 'emacs-minor-version))
(string-match "^[0-9]+\\.\\([0-9]+\\)" emacs-version))
(setq emacs-minor-version
(string-to-int (substring emacs-version
(match-beginning 1) (match-end 1)))))
;;; Define a function to make it easier to check which version we're
;;; running.
(defun running-emacs-version-or-newer (major minor)
(or (> emacs-major-version major)
(and (= emacs-major-version major)
(>= emacs-minor-version minor))))
(cond ((and running-xemacs
(running-emacs-version-or-newer 19 6))
;;
;; Code requiring XEmacs/Lucid Emacs version 19.6 or newer goes here
;;
))
(cond ((>= emacs-major-version 19)
;;
;; Code for any vintage-19 emacs goes here
;;
))
(cond ((and (not running-xemacs)
(>= emacs-major-version 19))
;;
;; Code specific to FSF Emacs 19 (not XEmacs/Lucid Emacs) goes here
;;
))
(cond ((< emacs-major-version 19)
;;
;; Code specific to emacs 18 goes here
;;
))
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Customization of Specific Packages ;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;; Load gnuserv, which will allow you to connect to XEmacs sessions
;;; using `gnuclient'.
;; If you never run more than one XEmacs at a time, you might want to
;; always start gnuserv. Otherwise it is preferable to specify
;; `-f gnuserv-start' on the command line to one of the XEmacsen.
; (gnuserv-start)
;;; ********************
;;; Load efs, which uses the FTP protocol as a pseudo-filesystem.
;;; When this is loaded, the pathname syntax /user@host:/remote/path
;;; refers to files accessible through ftp.
;;;
(require 'dired)
;; compatible ange-ftp/efs initialization derived from code
;; from John Turner
;; As of 19.15, efs is bundled instead of ange-ftp.
;; NB: doesn't handle 20.0 properly, efs didn't appear until 20.1.
;;
;; The environment variable EMAIL_ADDRESS is used as the password
;; for access to anonymous ftp sites, if it is set. If not, one is
;; constructed using the environment variables USER and DOMAINNAME
;; (e.g. turner@lanl.gov), if set.
(if (and running-xemacs
(or (and (= emacs-major-version 20) (>= emacs-minor-version 1))
(and (= emacs-major-version 19) (>= emacs-minor-version 15))))
(progn
(message "Loading and configuring bundled packages... efs")
(require 'efs-auto)
(if (getenv "USER")
(setq efs-default-user (getenv "USER")))
(if (getenv "EMAIL_ADDRESS")
(setq efs-generate-anonymous-password (getenv "EMAIL_ADDRESS"))
(if (and (getenv "USER")
(getenv "DOMAINNAME"))
(setq efs-generate-anonymous-password
(concat (getenv "USER")"@"(getenv "DOMAINNAME")))))
(setq efs-auto-save 1))
(progn
(message "Loading and configuring bundled packages... ange-ftp")
(require 'ange-ftp)
(if (getenv "USER")
(setq ange-ftp-default-user (getenv "USER")))
(if (getenv "EMAIL_ADDRESS")
(setq ange-ftp-generate-anonymous-password (getenv "EMAIL_ADDRESS"))
(if (and (getenv "USER")
(getenv "DOMAINNAME"))
(setq ange-ftp-generate-anonymous-password
(concat (getenv "USER")"@"(getenv "DOMAINNAME")))))
(setq ange-ftp-auto-save 1)
)
)
;;; ********************
;;; Load the default-dir.el package which installs fancy handling
;;; of the initial contents in the minibuffer when reading
;;; file names.
(if (and running-xemacs
(or (and (= emacs-major-version 20) (>= emacs-minor-version 1))
(and (= emacs-major-version 19) (>= emacs-minor-version 15))))
(require 'default-dir))
;;; ********************
;;; Load the auto-save.el package, which lets you put all of your autosave
;;; files in one place, instead of scattering them around the file system.
;;;
(setq auto-save-directory (expand-file-name "~/autosave/")
auto-save-directory-fallback auto-save-directory
auto-save-hash-p nil
efs-auto-save t
efs-auto-save-remotely nil
;; now that we have auto-save-timeout, let's crank this up
;; for better interactive response.
auto-save-interval 2000
)
;; We load this afterwards because it checks to make sure the
;; auto-save-directory exists (creating it if not) when it's loaded.
(require 'auto-save)
;; This adds additional extensions which indicate files normally
;; handled by cc-mode.
(setq auto-mode-alist
(append '(("\\.C$" . c++-mode)
("\\.cc$" . c++-mode)
("\\.hh$" . c++-mode)
("\\.c$" . c-mode)
("\\.h$" . c-mode))
auto-mode-alist))
;;; ********************
;;; cc-mode (the mode you're in when editing C, C++, and Objective C files)
;; Tell cc-mode not to check for old-style (K&R) function declarations.
;; This speeds up indenting a lot.
(setq c-recognize-knr-p nil)
;; Change the indentation amount to 4 spaces instead of 2.
;; You have to do it in this complicated way because of the
;; strange way the cc-mode initializes the value of `c-basic-offset'.
(add-hook 'c-mode-hook (lambda () (setq c-basic-offset 4)))
;;; ********************
;;; Load a partial-completion mechanism, which makes minibuffer completion
;;; search multiple words instead of just prefixes; for example, the command
;;; `M-x byte-compile-and-load-file RET' can be abbreviated as `M-x b-c-a RET'
;;; because there are no other commands whose first three words begin with
;;; the letters `b', `c', and `a' respectively.
;;;
(load-library "completer")
;;; ********************
;;; Load crypt, which is a package for automatically decoding and reencoding
;;; files by various methods - for example, you can visit a .Z or .gz file,
;;; edit it, and have it automatically re-compressed when you save it again.
;;;
(setq crypt-encryption-type 'pgp ; default encryption mechanism
crypt-confirm-password t ; make sure new passwords are correct
;crypt-never-ever-decrypt t ; if you don't encrypt anything, set this to
; tell it not to assume that "binary" files
; are encrypted and require a password.
)
(require 'crypt)
;;; ********************
;;; Edebug is a source-level debugger for emacs-lisp programs.
;;;
(define-key emacs-lisp-mode-map "\C-xx" 'edebug-defun)
;;; ********************
;;; Font-Lock is a syntax-highlighting package. When it is enabled and you
;;; are editing a program, different parts of your program will appear in
;;; different fonts or colors. For example, with the code below, comments
;;; appear in red italics, function names in function definitions appear in
;;; blue bold, etc. The code below will cause font-lock to automatically be
;;; enabled when you edit C, C++, Emacs-Lisp, and many other kinds of
;;; programs.
;;;
;;; The "Options" menu has some commands for controlling this as well.
;;;
(cond (running-xemacs
;; If you want the default colors, you could do this:
;; (setq font-lock-use-default-fonts nil)
;; (setq font-lock-use-default-colors t)
;; but I want to specify my own colors, so I turn off all
;; default values.
(setq font-lock-use-default-fonts nil)
(setq font-lock-use-default-colors nil)
(require 'font-lock)
;; Mess around with the faces a bit. Note that you have
;; to change the font-lock-use-default-* variables *before*
;; loading font-lock, and wait till *after* loading font-lock
;; to customize the faces.
;; string face is green
(set-face-foreground 'font-lock-string-face "forest green")
;; comments are italic and red; doc strings are italic
;;
;; (I use copy-face instead of make-face-italic/make-face-bold
;; because the startup code does intelligent things to the
;; 'italic and 'bold faces to ensure that they are different
;; from the default face. For example, if the default face
;; is bold, then the 'bold face will be unbold.)
(copy-face 'italic 'font-lock-comment-face)
;; Underlining comments looks terrible on tty's
(set-face-underline-p 'font-lock-comment-face nil 'global 'tty)
(set-face-highlight-p 'font-lock-comment-face t 'global 'tty)
(copy-face 'font-lock-comment-face 'font-lock-doc-string-face)
(set-face-foreground 'font-lock-comment-face "red")
;; function names are bold and blue
(copy-face 'bold 'font-lock-function-name-face)
(set-face-foreground 'font-lock-function-name-face "blue")
;; misc. faces
(and (find-face 'font-lock-preprocessor-face) ; 19.13 and above
(copy-face 'bold 'font-lock-preprocessor-face))
(copy-face 'italic 'font-lock-type-face)
(copy-face 'bold 'font-lock-keyword-face)
))
;;; ********************
;;; fast-lock is a package which speeds up the highlighting of files
;;; by saving information about a font-locked buffer to a file and
;;; loading that information when the file is loaded again. This
;;; requires a little extra disk space be used.
;;;
;;; Normally fast-lock puts the cache file (the filename appended with
;;; .flc) in the same directory as the file it caches. You can
;;; specify an alternate directory to use by setting the variable
;;; fast-lock-cache-directories.
;; Let's use lazy-lock instead.
;;(add-hook 'font-lock-mode-hook 'turn-on-fast-lock)
;;(setq fast-lock-cache-directories '("/foo/bar/baz"))
;;; ********************
;;; lazy-lock is a package which speeds up the highlighting of files
;;; by doing it "on-the-fly" -- only the visible portion of the
;;; buffer is fontified. The results may not always be quite as
;;; accurate as using full font-lock or fast-lock, but it's *much*
;;; faster. No more annoying pauses when you load files.
(add-hook 'font-lock-mode-hook 'turn-on-lazy-lock)
;; I personally don't like "stealth mode" (where lazy-lock starts
;; fontifying in the background if you're idle for 30 seconds)
;; because it takes too long to wake up again on my piddly Sparc 1+.
(setq lazy-lock-stealth-time nil)
;;; ********************
;;; func-menu is a package that scans your source file for function
;;; definitions and makes a menubar entry that lets you jump to any
;;; particular function definition by selecting it from the menu. The
;;; following code turns this on for all of the recognized languages.
;;; Scanning the buffer takes some time, but not much.
;;;
;;; Send bug reports, enhancements etc to:
;;; David Hughes
;;;
(cond (running-xemacs
(require 'func-menu)
(define-key global-map 'f8 'function-menu)
(add-hook 'find-file-hooks 'fume-add-menubar-entry)
(define-key global-map "\C-cl" 'fume-list-functions)
(define-key global-map "\C-cg" 'fume-prompt-function-goto)
;; The Hyperbole information manager package uses (shift button2) and
;; (shift button3) to provide context-sensitive mouse keys. If you
;; use this next binding, it will conflict with Hyperbole's setup.
;; Choose another mouse key if you use Hyperbole.
(define-key global-map '(shift button3) 'mouse-function-menu)
;; For descriptions of the following user-customizable variables,
;; type C-h v
(setq fume-max-items 25
fume-fn-window-position 3
fume-auto-position-popup t
fume-display-in-modeline-p t
fume-menubar-menu-location "File"
fume-buffer-name "*Function List*"
fume-no-prompt-on-valid-default nil)
))
;;; ********************
;;; MH is a mail-reading system from the Rand Corporation that relies on a
;;; number of external filter programs (which do not come with emacs.)
;;; Emacs provides a nice front-end onto MH, called "mh-e".
;;;
;; Bindings that let you send or read mail using MH
;(global-set-key "\C-xm" 'mh-smail)
;(global-set-key "\C-x4m" 'mh-smail-other-window)
;(global-set-key "\C-cr" 'mh-rmail)
;; Customization of MH behavior.
(setq mh-delete-yanked-msg-window t)
(setq mh-yank-from-start-of-msg 'body)
(setq mh-summary-height 11)
;; Use lines like the following if your version of MH
;; is in a special place.
;(setq mh-progs "/usr/dist/pkgs/mh/bin.svr4/")
;(setq mh-lib "/usr/dist/pkgs/mh/lib.svr4/")
;;; ********************
;;; resize-minibuffer-mode makes the minibuffer automatically
;;; resize as necessary when it's too big to hold its contents.
(autoload 'resize-minibuffer-mode "rsz-minibuf" nil t)
(resize-minibuffer-mode)
(setq resize-minibuffer-window-exactly nil)
;;; ********************
;;; W3 is a browser for the World Wide Web, and takes advantage of the very
;;; latest redisplay features in XEmacs. You can access it simply by typing
;;; 'M-x w3'; however, if you're unlucky enough to be on a machine that is
;;; behind a firewall, you will have to do something like this first:
;(setq w3-use-telnet t
; ;;
; ;; If the Telnet program you use to access the outside world is
; ;; not called "telnet", specify its name like this.
; w3-telnet-prog "itelnet"
; ;;
; ;; If your Telnet program adds lines of junk at the beginning
; ;; of the session, specify the number of lines here.
; w3-telnet-header-length 4
; )
Thanks to :
http://www.uluga.ubuntuforums.org/showthread.php?t=1375454
http://www.dotemacs.de/dotfiles/sample.emacs.html
http://www.dotemacs.de/dotfiles/BenjaminRutt.emacs.html
you can't find your .emacs file anywhere in your system. You've already looked in you home directory and it's nowhere to be found. Could somebody help me out?
So the solution is here.
There are two main versions of emacs - emacs and Xemacs and I don't think that either of them creates a .emacs file automatically - you have to create your own.
So what do you put in your .emacs? There are lots of examples of .emacs's that you can google for on the net, but here's one to start you off...
http://www.dotemacs.de/dotfiles/sample.emacs.html
...just save it to .emacs in your home directory.
If you have Xemacs installed, they don't use a file called .emacs but create a directory called .xemacs. Inside that directory you create a file called custom.el and that functions as your .emacs.
location of .emacs file :
/home/yourname/.emacs
eg: /home/siva/.emacs
Then add according to your need of your choice in emacs and save the file
sample .emacs file
;; -*- Mode: Emacs-Lisp -*-
;;; This is a sample .emacs file.
;;;
;;; The .emacs file, which should reside in your home directory, allows you to
;;; customize the behavior of Emacs. In general, changes to your .emacs file
;;; will not take effect until the next time you start up Emacs. You can load
;;; it explicitly with `M-x load-file RET ~/.emacs RET'.
;;;
;;; There is a great deal of documentation on customization in the Emacs
;;; manual. You can read this manual with the online Info browser: type
;;; `C-h i' or select "Emacs Info" from the "Help" menu.
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Basic Customization ;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Enable the command `narrow-to-region' ("C-x n n"), a useful
;; command, but possibly confusing to a new user, so it's disabled by
;; default.
(put 'narrow-to-region 'disabled nil)
;;; Define a variable to indicate whether we're running XEmacs/Lucid Emacs.
;;; (You do not have to defvar a global variable before using it --
;;; you can just call `setq' directly like we do for `emacs-major-version'
;;; below. It's clearer this way, though.)
(defvar running-xemacs (string-match "XEmacs\\|Lucid" emacs-version))
;; Make the sequence "C-x w" execute the `what-line' command,
;; which prints the current line number in the echo area.
(global-set-key "\C-xw" 'what-line)
;; set up the function keys to do common tasks to reduce Emacs pinky
;; and such.
;; Make F1 invoke help
(global-set-key [f1] 'help-command)
;; Make F2 be `undo'
(global-set-key [f2] 'undo)
;; Make F3 be `find-file'
;; Note: it does not currently work to say
;; (global-set-key 'f3 "\C-x\C-f")
;; The reason is that macros can't do interactive things properly.
;; This is an extremely longstanding bug in Emacs. Eventually,
;; it will be fixed. (Hopefully ..)
(global-set-key [f3] 'find-file)
;; Make F4 be "mark", F5 be "copy", F6 be "paste"
;; Note that you can set a key sequence either to a command or to another
;; key sequence.
(global-set-key [f4] 'set-mark-command)
(global-set-key [f5] "\M-w")
(global-set-key [f6] "\C-y")
;; Shift-F4 is "pop mark off of stack"
(global-set-key [(shift f4)] (lambda () (interactive) (set-mark-command t)))
;; Make F7 be `save-buffer'
(global-set-key [f7] 'save-buffer)
;; Make F8 be "start macro", F9 be "end macro", F10 be "execute macro"
(global-set-key [f8] 'start-kbd-macro)
(global-set-key [f9] 'end-kbd-macro)
(global-set-key [f10] 'call-last-kbd-macro)
;; Here's an alternative binding if you don't use keyboard macros:
;; Make F8 be `save-buffer' followed by `delete-window'.
;;(global-set-key 'f8 "\C-x\C-s\C-x0")
;; If you prefer delete to actually delete forward then you want to
;; uncomment the next line (or use `Customize' to customize this).
;; (setq delete-key-deletes-forward t)
(cond (running-xemacs
;;
;; Code for any version of XEmacs/Lucid Emacs goes here
;;
;; Change the values of some variables.
;; (t means true; nil means false.)
;;
;; Use the "Describe Variable..." option on the "Help" menu
;; to find out what these variables mean.
(setq find-file-use-truenames nil
find-file-compare-truenames t
minibuffer-confirm-incomplete t
complex-buffers-menu-p t
next-line-add-newlines nil
mail-yank-prefix "> "
kill-whole-line t
)
;; When running ispell, consider all 1-3 character words as correct.
(setq ispell-extra-args '("-W" "3"))
(cond ((or (not (fboundp 'device-type))
(equal (device-type) 'x))
;; Code which applies only when running emacs under X goes here.
;; (We check whether the function `device-type' exists
;; before using it. In versions before 19.12, there
;; was no such function. If it doesn't exist, we
;; simply assume we're running under X -- versions before
;; 19.12 only supported X.)
;; Remove the binding of C-x C-c, which normally exits emacs.
;; It's easy to hit this by mistake, and that can be annoying.
;; Under X, you can always quit with the "Exit Emacs" option on
;; the File menu.
(global-set-key "\C-x\C-c" nil)
;; Uncomment this to enable "sticky modifier keys" in 19.13
;; and up. With sticky modifier keys enabled, you can
;; press and release a modifier key before pressing the
;; key to be modified, like how the ESC key works always.
;; If you hold the modifier key down, however, you still
;; get the standard behavior. I personally think this
;; is the best thing since sliced bread (and a *major*
;; win when it comes to reducing Emacs pinky), but it's
;; disorienting at first so I'm not enabling it here by
;; default.
;;(setq modifier-keys-are-sticky t)
;; This changes the variable which controls the text that goes
;; in the top window title bar. (However, it is not changed
;; unless it currently has the default value, to avoid
;; interfering with a -wn command line argument I may have
;; started emacs with.)
(if (equal frame-title-format "%S: %b")
(setq frame-title-format
(concat "%S: " invocation-directory invocation-name
" [" emacs-version "]"
(if nil ; (getenv "NCD")
""
" %b"))))
;; If we're running on display 0, load some nifty sounds that
;; will replace the default beep. But if we're running on a
;; display other than 0, which probably means my NCD X terminal,
;; which can't play digitized sounds, do two things: reduce the
;; beep volume a bit, and change the pitch of the sound that is
;; made for "no completions."
;;
;; (Note that sampled sounds only work if XEmacs was compiled
;; with sound support, and we're running on the console of a
;; Sparc, HP, or SGI machine, or on a machine which has a
;; NetAudio server; otherwise, you just get the standard beep.)
;;
;; (Note further that changing the pitch and duration of the
;; standard beep only works with some X servers; many servers
;; completely ignore those parameters.)
;;
(cond ((string-match ":0" (getenv "DISPLAY"))
(load-default-sounds))
(t
(setq bell-volume 40)
(setq sound-alist
(append sound-alist '((no-completion :pitch 500))))
))
;; Make `C-x C-m' and `C-x RET' be different (since I tend
;; to type the latter by accident sometimes.)
(define-key global-map [(control x) return] nil)
;; Change the pointer used when the mouse is over a modeline
(set-glyph-image modeline-pointer-glyph "leftbutton")
;; Change the continuation glyph face so it stands out more
(and (fboundp 'set-glyph-property)
(boundp 'continuation-glyph)
(set-glyph-property continuation-glyph 'face 'bold))
;; Change the pointer used during garbage collection.
;;
;; Note that this pointer image is rather large as pointers go,
;; and so it won't work on some X servers (such as the MIT
;; R5 Sun server) because servers may have lamentably small
;; upper limits on pointer size.
;;(if (featurep 'xpm)
;; (set-glyph-image gc-pointer-glyph
;; (expand-file-name "trash.xpm" data-directory)))
;; Here's another way to do that: it first tries to load the
;; pointer once and traps the error, just to see if it's
;; possible to load that pointer on this system; if it is,
;; then it sets gc-pointer-glyph, because we know that
;; will work. Otherwise, it doesn't change that variable
;; because we know it will just cause some error messages.
(if (featurep 'xpm)
(let ((file (expand-file-name "recycle.xpm" data-directory)))
(if (condition-case error
;; check to make sure we can use the pointer.
(make-image-instance file nil
'(pointer))
(error nil)) ; returns nil if an error occurred.
(set-glyph-image gc-pointer-glyph file))))
(when (featurep 'menubar)
;; Add `dired' to the File menu
(add-menu-button '("File") ["Edit Directory" dired t])
;; Here's a way to add scrollbar-like buttons to the menubar
(add-menu-button nil ["Top" beginning-of-buffer t])
(add-menu-button nil ["<<<" scroll-down t])
(add-menu-button nil [" . " recenter t])
(add-menu-button nil [">>>" scroll-up t])
(add-menu-button nil ["Bot" end-of-buffer t]))
;; Change the behavior of mouse button 2 (which is normally
;; bound to `mouse-yank'), so that it inserts the selected text
;; at point (where the text cursor is), instead of at the
;; position clicked.
;;
;; Note that you can find out what a particular key sequence or
;; mouse button does by using the "Describe Key..." option on
;; the Help menu.
(setq mouse-yank-at-point t)
;; When editing C code (and Lisp code and the like), I often
;; like to insert tabs into comments and such. It gets to be
;; a pain to always have to use `C-q TAB', so I set up a more
;; convenient binding. Note that this does not work in
;; TTY frames, where tab and shift-tab are indistinguishable.
(define-key global-map '(shift tab) 'self-insert-command)
;; LISPM bindings of Control-Shift-C and Control-Shift-E.
;; Note that "\C-C" means Control-C, not Control-Shift-C.
;; To specify shifted control characters, you must use the
;; more verbose syntax used here.
(define-key emacs-lisp-mode-map '(control C) 'compile-defun)
(define-key emacs-lisp-mode-map '(control E) 'eval-defun)
;; If you like the FSF Emacs binding of button3 (single-click
;; extends the selection, double-click kills the selection),
;; uncomment the following:
;; Under 19.13, the following is enough:
;(define-key global-map 'button3 'mouse-track-adjust)
;; But under 19.12, you need this:
;(define-key global-map 'button3
; (lambda (event)
; (interactive "e")
; (let ((default-mouse-track-adjust t))
; (mouse-track event))))
;; Under both 19.12 and 19.13, you also need this:
;(add-hook 'mouse-track-click-hook
; (lambda (event count)
; (if (or (/= (event-button event) 3)
; (/= count 2))
; nil ;; do the normal operation
; (kill-region (point) (mark))
; t ;; don't do the normal operations.
; )))
))
))
;; Oh, and here's a cute hack you might want to put in the sample .emacs
;; file: it changes the color of the window if it's not on the local
;; machine, or if it's running as root:
;; local emacs background: whitesmoke
;; remote emacs background: palegreen1
;; root emacs background: coral2
(cond
((and (string-match "XEmacs" emacs-version)
(eq window-system 'x)
(boundp 'emacs-major-version)
(= emacs-major-version 19)
(>= emacs-minor-version 12))
(let* ((root-p (eq 0 (user-uid)))
(dpy (or (getenv "DISPLAY") ""))
(remote-p (not
(or (string-match "^\\(\\|unix\\|localhost\\):" dpy)
(let ((s (system-name)))
(if (string-match "\\.\\(netscape\\|mcom\\)\\.com" s)
(setq s (substring s 0 (match-beginning 0))))
(string-match (concat "^" (regexp-quote s)) dpy)))))
(bg (cond (root-p "coral2")
(remote-p "palegreen1")
(t nil))))
(cond (bg
(let ((def (color-name (face-background 'default)))
(faces (face-list)))
(while faces
(let ((obg (face-background (car faces))))
(if (and obg (equal def (color-name obg)))
(set-face-background (car faces) bg)))
(setq faces (cdr faces)))))))))
;;; Older versions of emacs did not have these variables
;;; (emacs-major-version and emacs-minor-version.)
;;; Let's define them if they're not around, since they make
;;; it much easier to conditionalize on the emacs version.
(if (and (not (boundp 'emacs-major-version))
(string-match "^[0-9]+" emacs-version))
(setq emacs-major-version
(string-to-int (substring emacs-version
(match-beginning 0) (match-end 0)))))
(if (and (not (boundp 'emacs-minor-version))
(string-match "^[0-9]+\\.\\([0-9]+\\)" emacs-version))
(setq emacs-minor-version
(string-to-int (substring emacs-version
(match-beginning 1) (match-end 1)))))
;;; Define a function to make it easier to check which version we're
;;; running.
(defun running-emacs-version-or-newer (major minor)
(or (> emacs-major-version major)
(and (= emacs-major-version major)
(>= emacs-minor-version minor))))
(cond ((and running-xemacs
(running-emacs-version-or-newer 19 6))
;;
;; Code requiring XEmacs/Lucid Emacs version 19.6 or newer goes here
;;
))
(cond ((>= emacs-major-version 19)
;;
;; Code for any vintage-19 emacs goes here
;;
))
(cond ((and (not running-xemacs)
(>= emacs-major-version 19))
;;
;; Code specific to FSF Emacs 19 (not XEmacs/Lucid Emacs) goes here
;;
))
(cond ((< emacs-major-version 19)
;;
;; Code specific to emacs 18 goes here
;;
))
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Customization of Specific Packages ;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;; Load gnuserv, which will allow you to connect to XEmacs sessions
;;; using `gnuclient'.
;; If you never run more than one XEmacs at a time, you might want to
;; always start gnuserv. Otherwise it is preferable to specify
;; `-f gnuserv-start' on the command line to one of the XEmacsen.
; (gnuserv-start)
;;; ********************
;;; Load efs, which uses the FTP protocol as a pseudo-filesystem.
;;; When this is loaded, the pathname syntax /user@host:/remote/path
;;; refers to files accessible through ftp.
;;;
(require 'dired)
;; compatible ange-ftp/efs initialization derived from code
;; from John Turner
;; As of 19.15, efs is bundled instead of ange-ftp.
;; NB: doesn't handle 20.0 properly, efs didn't appear until 20.1.
;;
;; The environment variable EMAIL_ADDRESS is used as the password
;; for access to anonymous ftp sites, if it is set. If not, one is
;; constructed using the environment variables USER and DOMAINNAME
;; (e.g. turner@lanl.gov), if set.
(if (and running-xemacs
(or (and (= emacs-major-version 20) (>= emacs-minor-version 1))
(and (= emacs-major-version 19) (>= emacs-minor-version 15))))
(progn
(message "Loading and configuring bundled packages... efs")
(require 'efs-auto)
(if (getenv "USER")
(setq efs-default-user (getenv "USER")))
(if (getenv "EMAIL_ADDRESS")
(setq efs-generate-anonymous-password (getenv "EMAIL_ADDRESS"))
(if (and (getenv "USER")
(getenv "DOMAINNAME"))
(setq efs-generate-anonymous-password
(concat (getenv "USER")"@"(getenv "DOMAINNAME")))))
(setq efs-auto-save 1))
(progn
(message "Loading and configuring bundled packages... ange-ftp")
(require 'ange-ftp)
(if (getenv "USER")
(setq ange-ftp-default-user (getenv "USER")))
(if (getenv "EMAIL_ADDRESS")
(setq ange-ftp-generate-anonymous-password (getenv "EMAIL_ADDRESS"))
(if (and (getenv "USER")
(getenv "DOMAINNAME"))
(setq ange-ftp-generate-anonymous-password
(concat (getenv "USER")"@"(getenv "DOMAINNAME")))))
(setq ange-ftp-auto-save 1)
)
)
;;; ********************
;;; Load the default-dir.el package which installs fancy handling
;;; of the initial contents in the minibuffer when reading
;;; file names.
(if (and running-xemacs
(or (and (= emacs-major-version 20) (>= emacs-minor-version 1))
(and (= emacs-major-version 19) (>= emacs-minor-version 15))))
(require 'default-dir))
;;; ********************
;;; Load the auto-save.el package, which lets you put all of your autosave
;;; files in one place, instead of scattering them around the file system.
;;;
(setq auto-save-directory (expand-file-name "~/autosave/")
auto-save-directory-fallback auto-save-directory
auto-save-hash-p nil
efs-auto-save t
efs-auto-save-remotely nil
;; now that we have auto-save-timeout, let's crank this up
;; for better interactive response.
auto-save-interval 2000
)
;; We load this afterwards because it checks to make sure the
;; auto-save-directory exists (creating it if not) when it's loaded.
(require 'auto-save)
;; This adds additional extensions which indicate files normally
;; handled by cc-mode.
(setq auto-mode-alist
(append '(("\\.C$" . c++-mode)
("\\.cc$" . c++-mode)
("\\.hh$" . c++-mode)
("\\.c$" . c-mode)
("\\.h$" . c-mode))
auto-mode-alist))
;;; ********************
;;; cc-mode (the mode you're in when editing C, C++, and Objective C files)
;; Tell cc-mode not to check for old-style (K&R) function declarations.
;; This speeds up indenting a lot.
(setq c-recognize-knr-p nil)
;; Change the indentation amount to 4 spaces instead of 2.
;; You have to do it in this complicated way because of the
;; strange way the cc-mode initializes the value of `c-basic-offset'.
(add-hook 'c-mode-hook (lambda () (setq c-basic-offset 4)))
;;; ********************
;;; Load a partial-completion mechanism, which makes minibuffer completion
;;; search multiple words instead of just prefixes; for example, the command
;;; `M-x byte-compile-and-load-file RET' can be abbreviated as `M-x b-c-a RET'
;;; because there are no other commands whose first three words begin with
;;; the letters `b', `c', and `a' respectively.
;;;
(load-library "completer")
;;; ********************
;;; Load crypt, which is a package for automatically decoding and reencoding
;;; files by various methods - for example, you can visit a .Z or .gz file,
;;; edit it, and have it automatically re-compressed when you save it again.
;;;
(setq crypt-encryption-type 'pgp ; default encryption mechanism
crypt-confirm-password t ; make sure new passwords are correct
;crypt-never-ever-decrypt t ; if you don't encrypt anything, set this to
; tell it not to assume that "binary" files
; are encrypted and require a password.
)
(require 'crypt)
;;; ********************
;;; Edebug is a source-level debugger for emacs-lisp programs.
;;;
(define-key emacs-lisp-mode-map "\C-xx" 'edebug-defun)
;;; ********************
;;; Font-Lock is a syntax-highlighting package. When it is enabled and you
;;; are editing a program, different parts of your program will appear in
;;; different fonts or colors. For example, with the code below, comments
;;; appear in red italics, function names in function definitions appear in
;;; blue bold, etc. The code below will cause font-lock to automatically be
;;; enabled when you edit C, C++, Emacs-Lisp, and many other kinds of
;;; programs.
;;;
;;; The "Options" menu has some commands for controlling this as well.
;;;
(cond (running-xemacs
;; If you want the default colors, you could do this:
;; (setq font-lock-use-default-fonts nil)
;; (setq font-lock-use-default-colors t)
;; but I want to specify my own colors, so I turn off all
;; default values.
(setq font-lock-use-default-fonts nil)
(setq font-lock-use-default-colors nil)
(require 'font-lock)
;; Mess around with the faces a bit. Note that you have
;; to change the font-lock-use-default-* variables *before*
;; loading font-lock, and wait till *after* loading font-lock
;; to customize the faces.
;; string face is green
(set-face-foreground 'font-lock-string-face "forest green")
;; comments are italic and red; doc strings are italic
;;
;; (I use copy-face instead of make-face-italic/make-face-bold
;; because the startup code does intelligent things to the
;; 'italic and 'bold faces to ensure that they are different
;; from the default face. For example, if the default face
;; is bold, then the 'bold face will be unbold.)
(copy-face 'italic 'font-lock-comment-face)
;; Underlining comments looks terrible on tty's
(set-face-underline-p 'font-lock-comment-face nil 'global 'tty)
(set-face-highlight-p 'font-lock-comment-face t 'global 'tty)
(copy-face 'font-lock-comment-face 'font-lock-doc-string-face)
(set-face-foreground 'font-lock-comment-face "red")
;; function names are bold and blue
(copy-face 'bold 'font-lock-function-name-face)
(set-face-foreground 'font-lock-function-name-face "blue")
;; misc. faces
(and (find-face 'font-lock-preprocessor-face) ; 19.13 and above
(copy-face 'bold 'font-lock-preprocessor-face))
(copy-face 'italic 'font-lock-type-face)
(copy-face 'bold 'font-lock-keyword-face)
))
;;; ********************
;;; fast-lock is a package which speeds up the highlighting of files
;;; by saving information about a font-locked buffer to a file and
;;; loading that information when the file is loaded again. This
;;; requires a little extra disk space be used.
;;;
;;; Normally fast-lock puts the cache file (the filename appended with
;;; .flc) in the same directory as the file it caches. You can
;;; specify an alternate directory to use by setting the variable
;;; fast-lock-cache-directories.
;; Let's use lazy-lock instead.
;;(add-hook 'font-lock-mode-hook 'turn-on-fast-lock)
;;(setq fast-lock-cache-directories '("/foo/bar/baz"))
;;; ********************
;;; lazy-lock is a package which speeds up the highlighting of files
;;; by doing it "on-the-fly" -- only the visible portion of the
;;; buffer is fontified. The results may not always be quite as
;;; accurate as using full font-lock or fast-lock, but it's *much*
;;; faster. No more annoying pauses when you load files.
(add-hook 'font-lock-mode-hook 'turn-on-lazy-lock)
;; I personally don't like "stealth mode" (where lazy-lock starts
;; fontifying in the background if you're idle for 30 seconds)
;; because it takes too long to wake up again on my piddly Sparc 1+.
(setq lazy-lock-stealth-time nil)
;;; ********************
;;; func-menu is a package that scans your source file for function
;;; definitions and makes a menubar entry that lets you jump to any
;;; particular function definition by selecting it from the menu. The
;;; following code turns this on for all of the recognized languages.
;;; Scanning the buffer takes some time, but not much.
;;;
;;; Send bug reports, enhancements etc to:
;;; David Hughes
;;;
(cond (running-xemacs
(require 'func-menu)
(define-key global-map 'f8 'function-menu)
(add-hook 'find-file-hooks 'fume-add-menubar-entry)
(define-key global-map "\C-cl" 'fume-list-functions)
(define-key global-map "\C-cg" 'fume-prompt-function-goto)
;; The Hyperbole information manager package uses (shift button2) and
;; (shift button3) to provide context-sensitive mouse keys. If you
;; use this next binding, it will conflict with Hyperbole's setup.
;; Choose another mouse key if you use Hyperbole.
(define-key global-map '(shift button3) 'mouse-function-menu)
;; For descriptions of the following user-customizable variables,
;; type C-h v
(setq fume-max-items 25
fume-fn-window-position 3
fume-auto-position-popup t
fume-display-in-modeline-p t
fume-menubar-menu-location "File"
fume-buffer-name "*Function List*"
fume-no-prompt-on-valid-default nil)
))
;;; ********************
;;; MH is a mail-reading system from the Rand Corporation that relies on a
;;; number of external filter programs (which do not come with emacs.)
;;; Emacs provides a nice front-end onto MH, called "mh-e".
;;;
;; Bindings that let you send or read mail using MH
;(global-set-key "\C-xm" 'mh-smail)
;(global-set-key "\C-x4m" 'mh-smail-other-window)
;(global-set-key "\C-cr" 'mh-rmail)
;; Customization of MH behavior.
(setq mh-delete-yanked-msg-window t)
(setq mh-yank-from-start-of-msg 'body)
(setq mh-summary-height 11)
;; Use lines like the following if your version of MH
;; is in a special place.
;(setq mh-progs "/usr/dist/pkgs/mh/bin.svr4/")
;(setq mh-lib "/usr/dist/pkgs/mh/lib.svr4/")
;;; ********************
;;; resize-minibuffer-mode makes the minibuffer automatically
;;; resize as necessary when it's too big to hold its contents.
(autoload 'resize-minibuffer-mode "rsz-minibuf" nil t)
(resize-minibuffer-mode)
(setq resize-minibuffer-window-exactly nil)
;;; ********************
;;; W3 is a browser for the World Wide Web, and takes advantage of the very
;;; latest redisplay features in XEmacs. You can access it simply by typing
;;; 'M-x w3'; however, if you're unlucky enough to be on a machine that is
;;; behind a firewall, you will have to do something like this first:
;(setq w3-use-telnet t
; ;;
; ;; If the Telnet program you use to access the outside world is
; ;; not called "telnet", specify its name like this.
; w3-telnet-prog "itelnet"
; ;;
; ;; If your Telnet program adds lines of junk at the beginning
; ;; of the session, specify the number of lines here.
; w3-telnet-header-length 4
; )
Thanks to :
http://www.uluga.ubuntuforums.org/showthread.php?t=1375454
http://www.dotemacs.de/dotfiles/sample.emacs.html
http://www.dotemacs.de/dotfiles/BenjaminRutt.emacs.html
Wednesday, May 12, 2010
Hangman (game)
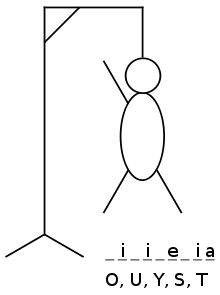
Hangman is a paper and pencil guessing game for two or more players. One player thinks of a word and the other tries to guess it by suggesting letters.
_______
|/ |
| O
| \|/
| |
| / \
__|_________
The word to guess is represented by a row of dashes, giving the number of letters. If the guessing player suggests a letter which occurs in the word, the other player writes it in all its correct positions. If the suggested letter does not occur in the word, the other player draws one element of the hangman diagram as a tally mark. The game is over when:
An example game in progress; the answer is Wikipedia.
* The guessing player completes the word, or guesses the whole word correctly
* The other player completes the diagram:
This diagram is, in fact, designed to look like a hanging man. Although debates have arisen about the questionable taste of this picture, it is still in use today. A common alternative for teachers is to draw an apple tree with ten apples, erasing or crossing out the apples as the guesses are used up.
The exact nature of the diagram differs; some players draw the gallows before play and draw parts of the man's body (traditionally the head, then the torso, then the left arm, then the right arm, then the left leg, then the right leg). Some players begin with no diagram at all, and drawing the individual elements of the gallows as part of the game, effectively giving the guessing players more chances. The amount of detail on the man can also vary, affecting the number of chances. Many players include a face on the head, either all at once or one feature at a time.
Some modifications to game play, such as "buying a vowel", result from the U.S. television game show Wheel of Fortune, created by Merv Griffin.
My -- Hangman C programming code
------------------------------------------------
#include
#include
#define len 8
int try1(){
printf("---------------\n");
printf("failed \n");
printf("---------------\n");
}
int try2(){
static int i=9;
static int j=0;
i--;
printf("\t %d more chances \n",i);
j++;
prn_galg(j);
while( i == 0){
printf("Time out \n");
exit(-1);
}
}
int showlogo() {
printf("--------------------------------------------\n");
printf("| # # # # # #### # # # # # |\n");
printf("| # # # # ## # # ## ## # # ## # |\n");
printf("| #### ##### # # # # ## # # # ##### # # # |\n");
printf("| # # # # # ## # # # # # # # ## |\n");
printf("| # # # # # # ### # # # # # # |\n");
printf("--------------------------------------------\n\n");
}
void prn_galg(int i) {
switch (i) {
case 0 :
// printf("Amount of wrong letters: %d\n\n", i);
printf("\n");
printf("\n");
printf("\n");
printf("\n");
printf("\n");
printf("\n");
printf("____________\n\n");
break;
case 1 :
// printf("Amount of wrong letters: %d\n\n", i);
printf("\n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf("__|_________\n\n");
break;
case 2 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf("__|_________\n\n");
break;
case 3 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |/\n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf("__|_________\n\n");
break;
case 4 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |/ | \n");
printf(" | O \n");
printf(" |\n");
printf(" |\n");
printf(" |\n");
printf("__|_________\n\n");
break;
case 5 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |/ | \n");
printf(" | O \n");
printf(" | |\n");
printf(" | |\n");
printf(" |\n");
printf("__|_________\n\n");
break;
case 6 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |/ | \n");
printf(" | O \n");
printf(" | \\|\n");
printf(" | | \n");
printf(" |\n");
printf("__|_________\n\n");
break;
case 7 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |/ | \n");
printf(" | O \n");
printf(" | \\|/\n");
printf(" | | \n");
printf(" |\n");
printf("__|_________\n\n");
break;
case 8 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |/ | \n");
printf(" | O \n");
printf(" | \\|/\n");
printf(" | | \n");
printf(" | /\n");
printf("__|_________\n\n");
break;
case 9 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |/ | \n");
printf(" | O \n");
printf(" | \\|/\n");
printf(" | | \n");
printf(" | / \\\n");
printf("__|_________\n\n");
break;
case 10 :
// printf("Amount of wrong letters: %d\n\n", i);
printf(" _______\n");
printf(" |/ | \n");
printf(" | X \n");
printf(" | \\|/\n");
printf(" | | \n");
printf(" | / \\\n");
printf("__|_________\n\n");
break;
}
}
int main(){
/* here in "hangman" to be found is "h _ _ g _ _ n" */
char h[len] = "hangman";
char c[len];
stop :
showlogo();
printf("**Welcome to Hangman Code**\n");
printf("word to find is h _ _ g _ _ n\n");
// printf(" Find H 1 2 G 3 4 N \n");
printf("Press 'e' for quit \n");
printf("Try for the 1 letter: \n");
c[0] = getchar();
if(c[0]== 'e'){
exit(-1);
}
try2();
while(c[0] == h[1]){ // A
c[1] = getchar();
while (c[1] == h[2]){ // N
c[2] = getchar();
while (c[2] == h[4]){ // M
c[3] = getchar();
while (c[3] == h[5]){ //N
printf("Hurray you have completed \n");
printf("****************************************\n");
printf("****************************************\n");
printf("Completed letters are %c%c%c%c%c%c%c \n",h[0],h[1],h[2],h[3],h[4],h[5],h[6]);
printf("****************************************\n");
printf("****************************************\n");
printf("Success you have made the Hangman code \n");
goto stop;
}
try2();
printf("Completed letters are %c%c%c%c%c _ n \n",h[0],h[1],h[2],h[3],h[4]);
printf("Try for the 4 letter: \n");
}
try2();
printf("Completed letters are %c%c%cg _ _ n \n",h[0],h[1],h[2]);
printf("Try for the 3 letter: \n");
}
try2();
printf("Completed letters are %c%c _ g _ _ n \n",h[0],h[1]);
printf("Try for the 2 letter :\n");
}
{
goto stop;
}
return 0;
}
// this is a sample base code i have written . Still more efficient code will be released // as soon as possible.
Thanks to
wikipedia
http://en.wikipedia.org/wiki/Hangman_(game)
www.daniweb.com/code/post1157561.html
Tuesday, May 4, 2010
C file input/output
The C programming language provides many standard library functions for file input and output. These functions make up the bulk of the C standard library header .
The I/O functionality of C is fairly low-level by modern standards; C abstracts all file operations into operations on streams of bytes, which may be "input streams" or "output streams". Unlike some earlier programming languages, C has no direct support for random-access data files; to read from a record in the middle of a file, the programmer must create a stream, seek to the middle of the file, and then read bytes in sequence from the stream.
The stream model of file I/O was popularized by the Unix operating system, which was developed concurrently with the C programming language itself. The vast majority of modern operating systems have inherited streams from Unix, and many languages in the C programming language family have inherited C's file I/O interface with few if any changes (for example, PHP). The C++ standard library reflects the "stream" concept in its syntax; see iostream.
Opening a file using fopen
A file is opened using fopen, which returns an I/O stream attached to the specified file or other device from which reading and writing can be done. If the function fails, it returns a null pointer.
The related C library function freopen performs the same operation after first closing any open stream associated with its parameters.
They are defined as
FILE *fopen(const char *path, const char *mode);
FILE *freopen(const char *path, const char *mode, FILE *fp);
The fopen function is essentially a slightly higher-level wrapper for the open system call of Unix operating systems. In the same way, fclose is often a thin wrapper for the Unix system call close, and the C FILE structure itself often corresponds to a Unix file descriptor. In POSIX environments, the fdopen function can be used to initialize a FILE structure from a file descriptor; however, file descriptors are a purely Unix concept not present in standard C.
The mode parameter to fopen and freopen must be a string that begins with one of the following sequences:
mode description starts..
r rb open for reading beginning
for further modes refer the man pages
Closing a stream using fclose
The fclose function takes one argument: a pointer to the FILE structure of the stream to close.
int fclose(FILE *fp);
Reading from a stream using fgetc
The fgetc function is used to read a character from a stream.
int fgetc(FILE *fp);
Writing a file using fwrite
fwrite is defined as
size_t fwrite (const void *array, size_t size, size_t count, FILE *stream);
fwrite function writes a block of data to the stream. It will write an array of count elements to the current position in the stream. For each element, it will write size bytes. The position indicator of the stream will be advanced by the number of bytes written successfully.
Writing a file using fwrite
fwrite is defined as
size_t fwrite (const void *array, size_t size, size_t count, FILE *stream);
fwrite function writes a block of data to the stream. It will write an array of count elements to the current position in the stream. For each element, it will write size bytes. The position indicator of the stream will be advanced by the number of bytes written successfully.
The function will return the number of elements written successfully. The return value will be equal to count if the write completes successfully. In case of a write error, the return value will be less than count.
The following program opens a file named new1.c, writes a string of characters to the file, then closes it.
Writing to a stream using fputc
The I/O functionality of C is fairly low-level by modern standards; C abstracts all file operations into operations on streams of bytes, which may be "input streams" or "output streams". Unlike some earlier programming languages, C has no direct support for random-access data files; to read from a record in the middle of a file, the programmer must create a stream, seek to the middle of the file, and then read bytes in sequence from the stream.
The stream model of file I/O was popularized by the Unix operating system, which was developed concurrently with the C programming language itself. The vast majority of modern operating systems have inherited streams from Unix, and many languages in the C programming language family have inherited C's file I/O interface with few if any changes (for example, PHP). The C++ standard library reflects the "stream" concept in its syntax; see iostream.
Opening a file using fopen
A file is opened using fopen, which returns an I/O stream attached to the specified file or other device from which reading and writing can be done. If the function fails, it returns a null pointer.
The related C library function freopen performs the same operation after first closing any open stream associated with its parameters.
They are defined as
FILE *fopen(const char *path, const char *mode);
FILE *freopen(const char *path, const char *mode, FILE *fp);
The fopen function is essentially a slightly higher-level wrapper for the open system call of Unix operating systems. In the same way, fclose is often a thin wrapper for the Unix system call close, and the C FILE structure itself often corresponds to a Unix file descriptor. In POSIX environments, the fdopen function can be used to initialize a FILE structure from a file descriptor; however, file descriptors are a purely Unix concept not present in standard C.
The mode parameter to fopen and freopen must be a string that begins with one of the following sequences:
mode description starts..
r rb open for reading beginning
for further modes refer the man pages
Closing a stream using fclose
The fclose function takes one argument: a pointer to the FILE structure of the stream to close.
int fclose(FILE *fp);
Reading from a stream using fgetc
The fgetc function is used to read a character from a stream.
int fgetc(FILE *fp);
Writing a file using fwrite
fwrite is defined as
size_t fwrite (const void *array, size_t size, size_t count, FILE *stream);
fwrite function writes a block of data to the stream. It will write an array of count elements to the current position in the stream. For each element, it will write size bytes. The position indicator of the stream will be advanced by the number of bytes written successfully.
Writing a file using fwrite
fwrite is defined as
size_t fwrite (const void *array, size_t size, size_t count, FILE *stream);
fwrite function writes a block of data to the stream. It will write an array of count elements to the current position in the stream. For each element, it will write size bytes. The position indicator of the stream will be advanced by the number of bytes written successfully.
The function will return the number of elements written successfully. The return value will be equal to count if the write completes successfully. In case of a write error, the return value will be less than count.
The following program opens a file named new1.c, writes a string of characters to the file, then closes it.
#include
#include
#include
int main(void)
{
FILE *fp;
size_t count;
const char *str = "hello\n";
fp = fopen("sample.txt", "w");
if(fp == NULL) {
perror("failed to open sample.txt");
return EXIT_FAILURE;
}
count = fwrite(str, 1, strlen(str), fp);
printf("Wrote %zu bytes. fclose(fp) %s.\n", count, fclose(fp) == 0 ? "succeeded" : "failed");
return EXIT_SUCCESS;
}
Writing to a stream using fputc
The fputc
function is used to write a character to a stream.
int fputc(int c, FILE *fp);
The parameter c
is silently converted to an unsigned char
before being output. If successful, fputc
returns the character written. If unsuccessful, fputc returns EOF
.
The standard macro putc
, also defined in
, behaves in almost the same way as fputc
, except that—being a macro—it may evaluate its arguments more than once.
The standard function putchar
, also defined in
, takes only the first argument, and is equivalent to putc(c, stdout)
where c
is that argument.
#include
#include
int main(void)
{
char buffer[5] = {0}; /* initialized to zeroes */
int i, rc;
FILE *fp = fopen("myfile", "rb");
if (fp == NULL) {
perror("Failed to open file \"myfile\"");
return EXIT_FAILURE;
}
for (i = 0; (rc = getc(fp)) != EOF && i < 5; buffer[i++] = rc)
;
fclose(fp);
if (i == 5) {
puts("The bytes read were...");
printf("%x %x %x %x %x\n", buffer[0], buffer[1], buffer[2], buffer[3], buffer[4]);
} else
fputs("There was an error reading the file.\n", stderr);
return EXIT_SUCCESS;
}
Subscribe to:
Posts (Atom)